How to Use AWS Lambda Functions and API Gateway with Python: A Comprehensive Guide
- Anthony
- Apr 7
- 4 min read
Updated: Apr 25
AWS Lambda and API Gateway are two powerful services that allow you to run code in the cloud and create scalable applications without the need to manage servers. This combination is particularly useful for developers looking to build serverless applications. In this guide, we’ll explore how to effectively use AWS Lambda functions and API Gateway using Python, providing step-by-step instructions and practical examples to help you get started.
Understanding AWS Lambda
AWS Lambda is a serverless compute service that automatically manages the underlying infrastructure for you. You simply upload your code, and Lambda takes care of everything required to run and scale your code with high availability. You can invoke Lambda functions from other AWS services or from any web application via HTTP requests.
Key Features of AWS Lambda
Event-driven: Automatically triggered by events, such as S3 uploads, DynamoDB updates, or API Gateway requests.
Automatic Scaling: Handles traffic spikes without any configuration; it can run multiple instances of your function in parallel.
Pay-as-you-go pricing: You only pay for the compute time you consume; there's no charge when your code isn’t running.
Setting Up Your AWS Account
Before you can use AWS Lambda, you need an AWS account. If you haven't created one yet, head over to the AWS Free Tier page and create an account.
Introduction to API Gateway
API Gateway is a fully managed service that simplifies the process of building, deploying, and managing APIs. It acts as a "front door" for any application that needs to access data, business logic, or functionality from your backend services.
Key Features of API Gateway
Support for multiple API protocols: REST, WebSocket, and HTTP APIs.
Built-in security: Options for API keys, AWS IAM permissions, and Usage Plans.
Monitoring and logging: Integrates with AWS CloudWatch to provide monitoring, logging, and alerts.
Creating Your First AWS Lambda Function
Step 1: Navigate to the Lambda Console
Log in to your AWS Management Console.
From the services, select Lambda.
Step 2: Create a New Function
Click on Create function.
Choose Author from scratch.
Provide a function name and select Python 3.x for the runtime.
Set permissions by creating a new role or using an existing one.
Step 3: Write Your Function Code
Inside the Lambda function editor, write a simple example function:
```python
def lambda_handler(event, context):
return {
'statusCode': 200,
'body': 'Hello from Lambda!'
}
```
Step 4: Test the Function
Click on Test in the console.
Create a new test event with default settings.
Click Test again to execute your function. You should see the output of your function on the right-hand side.
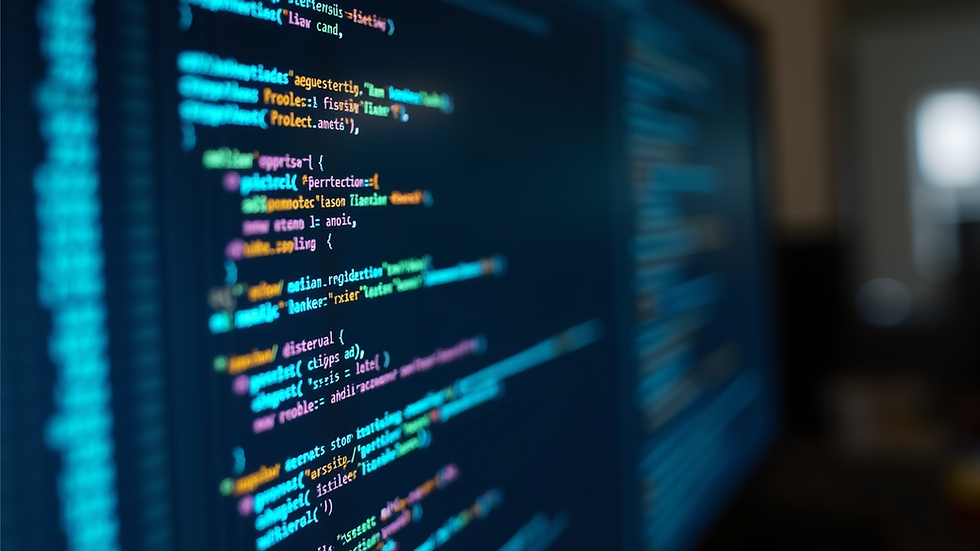
Setting Up API Gateway
After successfully creating your Lambda function, you’ll want to expose it via an API. This is where API Gateway comes in.
Step 1: Navigate to API Gateway Console
From the AWS Management Console, select API Gateway.
Step 2: Create a New API
Choose Create API.
Select HTTP API (for a simple setup).
Click Build.
Step 3: Configure Your API
Choose Add integration and select Lambda Function.
Select your previously created Lambda function from the dropdown menu.
Set the route by specifying a resource path, for example, `/hello`.
Click Create.
Step 4: Deploy Your API
Click Deployments in the left-hand menu.
Select Create and provide a stage name (e.g., `dev`).
Click Deploy. You will receive an endpoint URL.
Testing Your API
You can test your newly created API using various tools such as Postman or directly from your browser.
Paste the endpoint URL into your browser followed by `/hello`.
Example: `https://your-api-id.execute-api.region.amazonaws.com/dev/hello`
You should see the response:
```json
{
"statusCode": 200,
"body": "Hello from Lambda!"
}
```
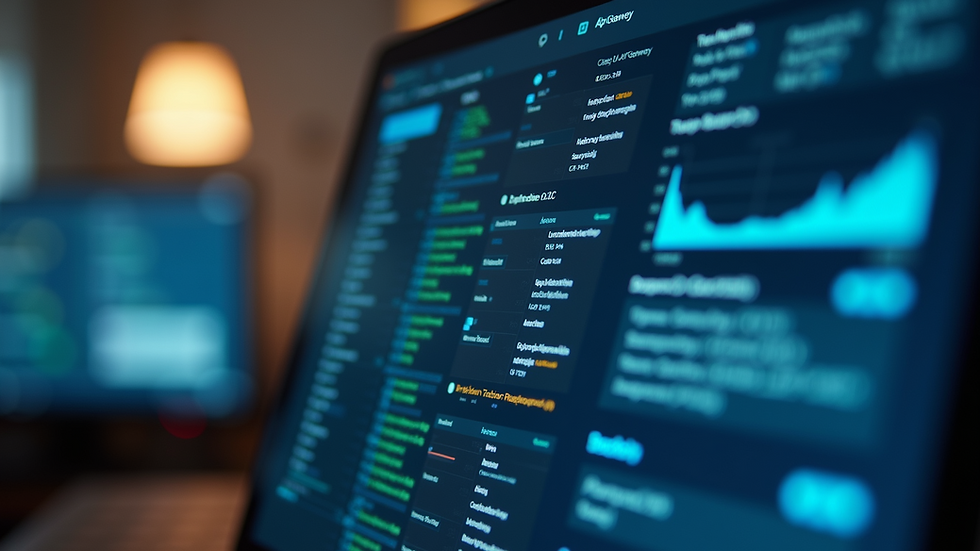
Handling Errors and Logging
Error handling and logging are essential components of any serverless application.
Setting Up Logging
AWS Lambda automatically integrates with CloudWatch to log all execution requests. You can view logs by navigating to CloudWatch in the AWS Console.
Error Handling in Lambda
You can enhance your lambda function to handle errors. Here’s an updated version of your function with basic error handling:
```python
def lambda_handler(event, context):
try:
return {
'statusCode': 200,
'body': 'Hello from Lambda!'
}
except Exception as e:
return {
'statusCode': 500,
'body': str(e)
}
```
Security Considerations
Securing your API should be a top priority. Here are some key measures:
Authentication and Authorization: Use IAM roles and policies to control access to your API Gateway.
Throttling and Rate Limiting: Set usage plans to limit the number of requests a single client can make.
CORS Configuration: If your API is accessed by a web application, ensure that you configure CORS settings properly.
Performance Best Practices
To optimize performance, consider the following:
Use Environment Variables: Store configurable variables in Lambda’s environment settings.
Optimize Deployment Package Size: Ensure that your deployment package does not contain unnecessary libraries or files.
Utilize Layers: Use Lambda layers to manage dependencies effectively.
Conclusion
Building serverless applications with AWS Lambda and API Gateway using Python is a powerful approach that can significantly reduce operating costs and increase scalability. By following the steps outlined in this guide, you should have a solid foundation to start building and deploying your serverless APIs.
Whether you are developing new applications or modernizing existing ones, embracing serverless architecture can lead to substantial benefits, including reduced time for innovation. With AWS continuously evolving and adding more features, there's never been a better time to dive into serverless technology.
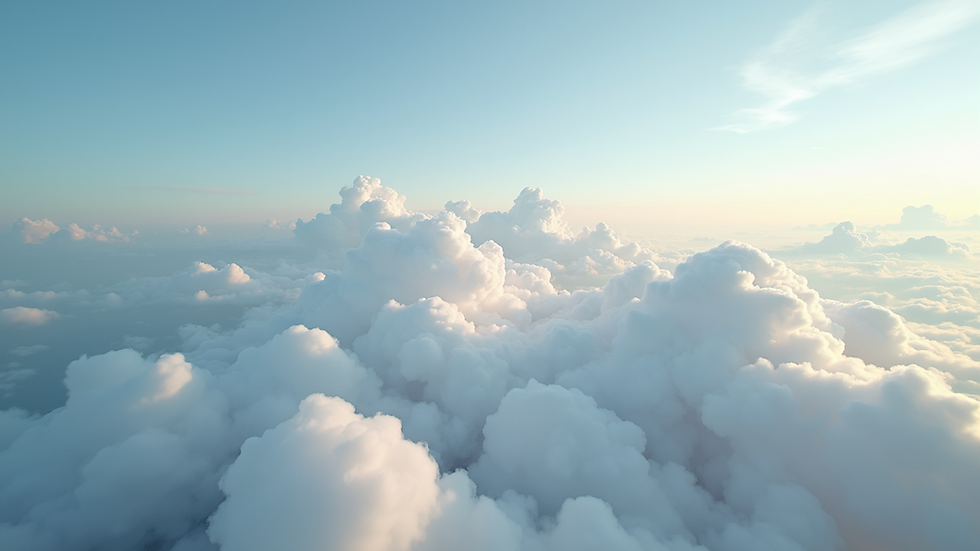
Now that you have an understanding of AWS Lambda functions and API Gateway, you can begin creating your own serverless solutions tailored to your specific business needs. Happy coding!
コメント